Get page views from Google Analytics using a Netlify Serverless function
Retrieving page views from Google Analytics using Netlify serverless functions
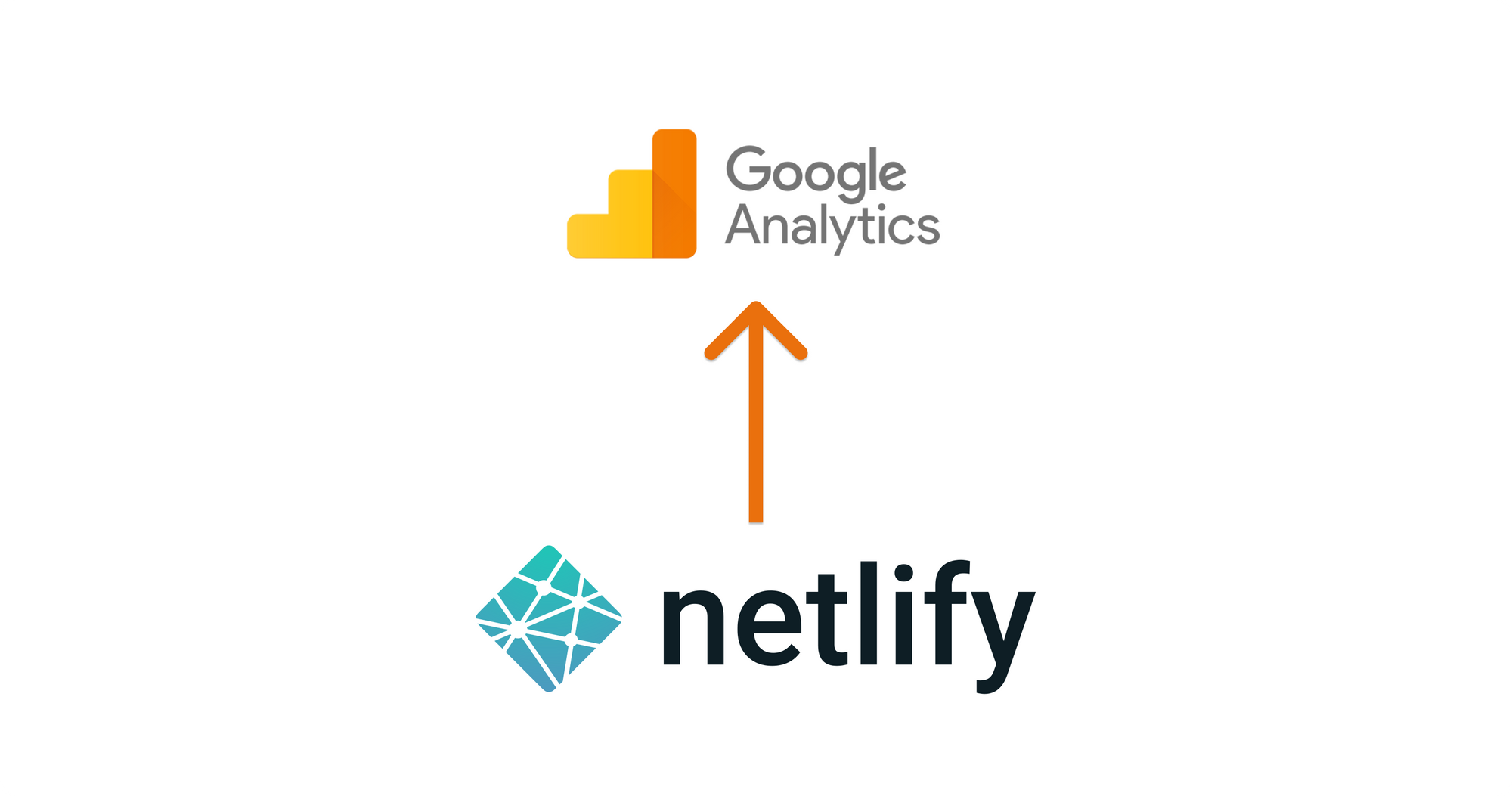
After getting this blog up and running I recently decided to add Google Analytics to it, to understand what content people were most interested in. At first this was amazing as I could see which blog posts were getting the most traction, but after a while it became a bit of a pain to log into my Google Analytics dashboard when all I wanted was to quickly see the page view count of a blog post.
Wouldn't it be easier to just go to my site and see the page view count displayed on each blog post? It most certainly would, and so that's what I set out to build. Since Google Analytics has a powerful API, all I really needed to do was call that API and retrieve my information, but ideally I wouldn't want to be making that call directly from this blog as that would mean exposing my API key and any other sensitive information to anyone who knows hows how to press F12.
TL;DR Here is the GitHub repository for the serverless function that I wrote for retrieving Google Analytics page views.
Since this blog is setup to be completely free (aside from the domain name), leveraging Netlify for the hosting, Cloudinary for images and Ghost for the content management system, I definitely wouldn't want to pay for running a back-end (especially one that was simply fetching stats from Google Analytics). And that's where Netlify functions come in. Netlify has a very generous free plan that allows 125k function executions and 100 hours execution time, a quota that this blog would never reach within 1 month, and this seemed like a perfect fit for what I needed.
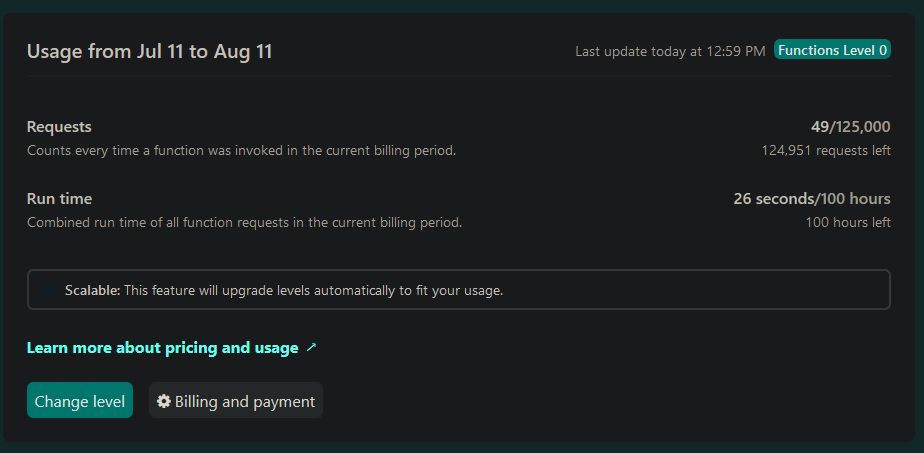
So let's dive into creating the serverless function for retrieving Google Analytics metrics.
Building the Google Analytics Serverless Function
As one does, I googled how to setup a Netlify function and came across this amazing blog post on the official Netlify blog which described how to run Express.js apps with Netlify functions.
Described in the above post is how AWS Lambda functions work at a high level, which is important since Netlify functions seems to be built ontop of that infrastructure. It also describes how the serverless-http npm package translates between the callback function and event object of Lambda functions to the req and res objects used in Express, allowing you to easily run Express.js apps as serverless functions. In fact , the guidance given should apply to any web framework built upon Node.js's http module.
Google Analytics authentication
In order to get access to your Google Analytics data you will need to authenticate when using the API. In order to do this, you will need to do the following:
- Create a new Google API Project.
- Enable the Google Analytics Reporting API.
- Create authentication credentials (Service account key) and generate a JSON key file.
- Add the created service account user to your Google Analytics instance in the User Management section.
- Retrieve the View ID for your website on Google Analytics.
The following blog posts have detailed descriptions on how to authenticate with google analytics and interacting with google analytics.
Once you've setup your Google API project with the correct permissions you can simply create a JWT object and call authorize on it, like this:
//create jwt object
const jwt = new google.auth.JWT(process.env.GA_CLIENT_EMAIL, null, process.env.GA_PRIVATE_KEY, 'https://www.googleapis.com/auth/analytics.readonly');
//authorize using jwt object
await jwt.authorize()
Fetching Google Analytics page views
As I said in the beginning of this post, Google Analytics has a powerful API exposing a vast array of metrics and dimensions which you can view here.
After identifying the metric and dimension you would like to extract from Google Analytics, you can use the Google API's npm package to retrieve the data you want.
/**
* Retrieves unique page views from Google Analytics
* @param {*} pagePath the path to filter from results
*/
async function getUniquePageViews(pagePath) {
const defaults = {
'auth': jwt,
'ids': 'ga:' + process.env.GA_VIEW_ID,
}
await jwt.authorize()
const metricRequestOptions = {
...defaults,
'start-date': startDate,
'end-date': 'today',
'metrics': 'ga:uniquePageviews',
'dimensions': 'ga:pagePath'
};
if (pagePath)
metricRequestOptions.filters = `ga:pagePath==${pagePath}`
const result = await google.analytics('v3').data.ga.get(metricRequestOptions)
return result.data.rows;
}
Executing the above request should return the page paths along with the number of unique page views
[
[
"/continuous-monitoring-using-flood-element-and-azure-devops/",
"46"
],
[
"/securing-traefik-dashboard-with-azure-ad/",
"80"
],
...
]
Once your serverless function is working correctly, you can then easily deploy it to Netlify.
The full source code can be found here.
Using this, I was able to get the unique page views to simply show up on my blog instead of having to go to the Google Analytics dashboard every time.

Endless possibilities
In this blog post we've gone through a very simple use case of how Netlify functions can be leveraged but the possibilities are truly endless.
How do you use serverless functions currently? Leave a comment and share your thoughts.